在移动应用开发中,上传图片到服务器是一个常见需求,无论是社交媒体应用、电子商务平台还是任何需要用户交互的应用,都可能需要将用户拍摄或选择的图片上传到服务器进行处理和存储,本文将详细介绍如何在Android应用中实现图片上传功能。

准备工作
1、环境搭建:确保你已经安装了Android Studio,并创建了一个新的Android项目。
2、权限配置:在AndroidManifest.xml
文件中添加必要的权限,特别是互联网访问权限和文件读写权限。
3、依赖管理:如果使用第三方库(如Retrofit或OkHttp)来处理HTTP请求,需要在build.gradle
文件中添加相应的依赖。
步骤一:获取图片
从相册选择图片:使用Intent来启动设备的图片选择器,允许用户从相册中选择一张图片。
拍照获取图片:使用相机Intent让用户拍摄一张新照片。
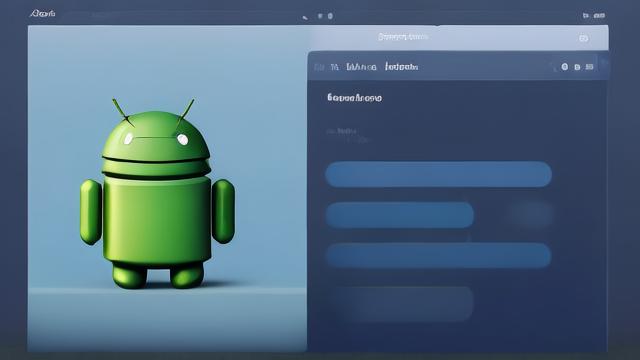
// 从相册选择图片 Intent pickPhoto = new Intent(Intent.ACTION_PICK, android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI); startActivityForResult(pickPhoto, PICK_PHOTO_CODE); // 拍照获取图片 Intent takePicture = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); startActivityForResult(takePicture, TAKE_PHOTO_CODE);
步骤二:压缩图片
由于直接上传原始图片可能会导致网络传输缓慢且消耗更多流量,因此通常需要先对图片进行压缩处理。
public static byte[] compressImage(String imagePath) throws IOException { Bitmap bitmap = BitmapFactory.decodeFile(imagePath); ByteArrayOutputStream baos = new ByteArrayOutputStream(); bitmap.compress(Bitmap.CompressFormat.JPEG, 50, baos); // 质量压缩为50% return baos.toByteArray(); }
步骤三:上传图片至服务器
使用HttpURLConnection:这是Java标准库提供的类,可以用来发送HTTP请求。
使用第三方库:如Retrofit或OkHttp,这些库提供了更简洁的API来处理网络通信。
使用HttpURLConnection上传图片
public void uploadImage(byte[] imageData) throws IOException { URL url = new URL("http://yourserver.com/upload"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setDoOutput(true); connection.setRequestMethod("POST"); connection.setRequestProperty("Content-Type", "image/jpeg"); try (OutputStream os = connection.getOutputStream()) { os.write(imageData); } finally { connection.disconnect(); } }
使用Retrofit上传图片
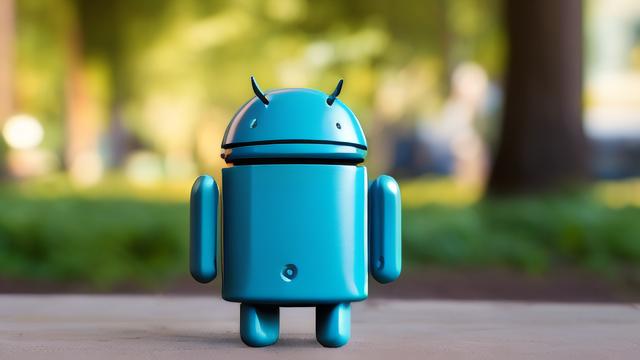
添加Retrofit依赖到你的build.gradle
文件中:
implementation 'com.squareup.retrofit2:retrofit:2.9.0' implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
定义一个接口来描述API端点:
public interface ApiService { @Multipart @POST("/upload") Call<Void> uploadImage(@Part MultipartBody.Part image); }
创建一个Retrofit实例并调用API:
Retrofit retrofit = new Retrofit.Builder() .baseUrl("http://yourserver.com/") .addConverterFactory(GsonConverterFactory.create()) .build(); ApiService apiService = retrofit.create(ApiService.class); MultipartBody.Part body = MultipartBody.Part.createFormData("file", filename, RequestBody.create(MediaType.parse("image/jpeg"), imageData)); Call<Void> call = apiService.uploadImage(body); call.enqueue(new Callback<Void>() { @Override public void onResponse(Call<Void> call, Response<Void> response) { if (response.isSuccessful()) { // 处理成功响应 } } @Override public void onFailure(Call<Void> call, Throwable t) { // 处理失败情况 } });
步骤四:处理服务器响应
根据服务器返回的数据格式(通常是JSON),解析响应并更新UI或执行其他逻辑,如果服务器返回了一个包含图片URL的JSON对象,你可以提取这个URL并在应用中使用它。
通过上述步骤,你可以在Android应用中实现一个完整的图片上传流程,包括从设备获取图片、压缩图片以及通过网络将图片上传到服务器,这个过程涉及到多个技术点,包括Intent的使用、Bitmap的处理、网络通信等,希望这篇指南能帮助你顺利完成任务!
到此,以上就是小编对于“android将图片上传至服务器”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。