Android工具类Toast自定义图片和文字
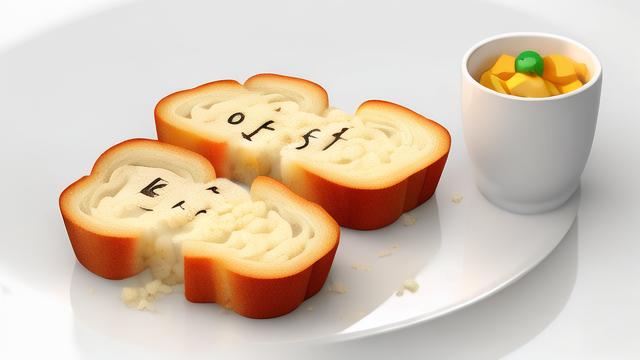
一、背景与目标
在Android开发中,Toast作为一种轻量级的提示工具被广泛使用,标准的Toast仅支持简单的文本显示,对于一些需要图文并茂提示的场景则显得力不从心,本文将详细介绍如何通过自定义Toast来实现同时显示图片和文字的功能。
二、基本用法与原理
简单用法
标准的Toast用法非常简单,适用于快速显示一条文本信息:
Toast.makeText(context, "Hello World", Toast.LENGTH_SHORT).show();
上述代码会在屏幕上显示一个短时间的Toast消息“Hello World”。
自定义显示位置
可以通过setGravity
方法设置Toast的显示位置:
toast.setGravity(Gravity.CENTER, 0, 0);
带图片效果
要实现带图片的Toast,需要用到自定义布局,以下是一个简单的自定义布局示例:
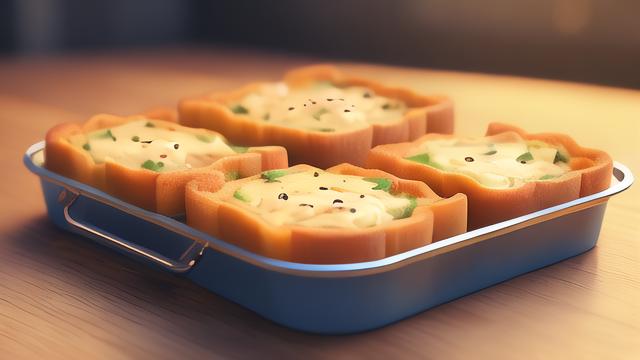
<!-res/layout/toast_view.xml --> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="#99919191" android:gravity="center" android:orientation="vertical"> <ImageView android:id="@+id/toast_image" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="20dp" android:scaleType="fitXY" /> <TextView android:id="@+id/toast_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="15sp" android:layout_marginBottom="20dp"/> </LinearLayout>
三、自定义Toast工具类实现
基础工具类
创建一个名为ToastUtils
的工具类,用于封装各种自定义Toast的实现:
public class ToastUtils { private static Context mContext = MyApplication.getContext(); public static void showToast(String message) { Toast.makeText(mContext, message, Toast.LENGTH_SHORT).show(); } }
带图片的Toast
实现一个带图片的Toast方法:
public static void showCustomImgToast(String text, int imgResId) { LayoutInflater inflater = LayoutInflater.from(mContext); View view = inflater.inflate(R.layout.toast_view, null); ImageView imageView = view.findViewById(R.id.toast_image); imageView.setImageResource(imgResId); TextView textView = view.findViewById(R.id.toast_text); textView.setText(text); Toast toast = new Toast(mContext); toast.setDuration(Toast.LENGTH_SHORT); toast.setView(view); toast.show(); }
不带图片的Toast
实现一个不带图片的Toast方法:
public static void showCustomToast(String text) { LayoutInflater inflater = LayoutInflater.from(mContext); View view = inflater.inflate(R.layout.toast_view, null); view.findViewById(R.id.toast_image).setVisibility(View.GONE); TextView textView = view.findViewById(R.id.toast_text); textView.setText(text); Toast toast = new Toast(mContext); toast.setDuration(Toast.LENGTH_SHORT); toast.setView(view); toast.show(); }
居中显示的Toast
实现一个居中显示的Toast方法:
public static void showCustomToastCenter(String text, int imgResId) { LayoutInflater inflater = LayoutInflater.from(mContext); View view = inflater.inflate(R.layout.toast_view, null); ImageView imageView = view.findViewById(R.id.toast_image); imageView.setImageResource(imgResId); TextView textView = view.findViewById(R.id.toast_text); textView.setText(text); Toast toast = new Toast(mContext); toast.setDuration(Toast.LENGTH_SHORT); toast.setView(view); toast.setGravity(Gravity.CENTER, 0, 0); toast.show(); }
四、完整示例与调用方式
1. 布局文件(res/layout/toast_view.xml)
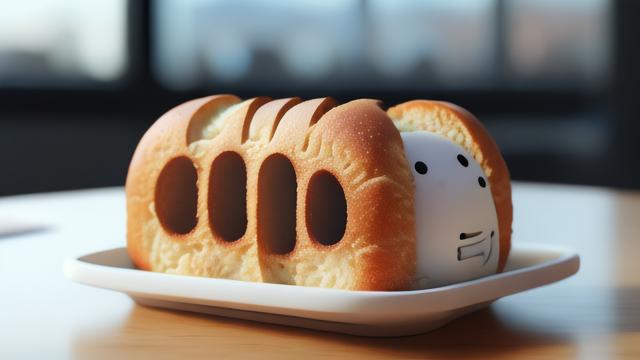
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="#99919191" android:gravity="center" android:orientation="vertical"> <ImageView android:id="@+id/toast_image" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="20dp" android:scaleType="fitXY" /> <TextView android:id="@+id/toast_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="15sp" android:layout_marginBottom="20dp"/> </LinearLayout>
2. 工具类实现(ToastUtils.java)
public class ToastUtils { private static Context mContext = MyApplication.getContext(); public static void showToast(String message) { Toast.makeText(mContext, message, Toast.LENGTH_SHORT).show(); } public static void showCustomImgToast(String text, int imgResId) { LayoutInflater inflater = LayoutInflater.from(mContext); View view = inflater.inflate(R.layout.toast_view, null); ImageView imageView = view.findViewById(R.id.toast_image); imageView.setImageResource(imgResId); TextView textView = view.findViewById(R.id.toast_text); textView.setText(text); Toast toast = new Toast(mContext); toast.setDuration(Toast.LENGTH_SHORT); toast.setView(view); toast.show(); } public static void showCustomToast(String text) { LayoutInflater inflater = LayoutInflater.from(mContext); View view = inflater.inflate(R.layout.toast_view, null); view.findViewById(R.id.toast_image).setVisibility(View.GONE); TextView textView = view.findViewById(R.id.toast_text); textView.setText(text); Toast toast = new Toast(mContext); toast.setDuration(Toast.LENGTH_SHORT); toast.setView(view); toast.show(); } public static void showCustomToastCenter(String text, int imgResId) { LayoutInflater inflater = LayoutInflater.from(mContext); View view = inflater.inflate(R.layout.toast_view, null); ImageView imageView = view.findViewById(R.id.toast_image); imageView.setImageResource(imgResId); TextView textView = view.findViewById(R.id.toast_text); textView.setText(text); Toast toast = new Toast(mContext); toast.setDuration(Toast.LENGTH_SHORT); toast.setView(view); toast.setGravity(Gravity.CENTER, 0, 0); toast.show(); } }
调用方式示例
// 显示普通Toast ToastUtils.showToast("This is a normal toast"); // 显示带图片的Toast ToastUtils.showCustomImgToast("This is an image toast", R.drawable.ic_launcher); // 显示不带图片的Toast ToastUtils.showCustomToast("This is a text-only toast"); // 显示居中的带图片Toast ToastUtils.showCustomToastCenter("This is a centered toast", R.drawable.ic_launcher);
五、归纳与扩展
通过自定义布局和工具类的方式,我们可以方便地在Android应用中实现各种复杂样式的Toast提示,这不仅提高了用户体验,也使得代码更加模块化和易于维护。
扩展功能建议
添加动画效果:可以为Toast添加出现和消失的动画效果,提升视觉效果。
支持更多自定义属性:例如字体颜色、背景颜色等,进一步增强自定义能力。
到此,以上就是小编对于“Android工具类Toast自定义图片和文字”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。