Android实现触摸移动的悬浮窗
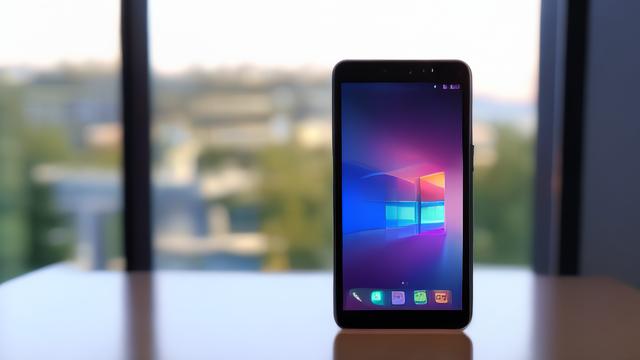
在Android应用开发中,悬浮窗(Floating Window)是一种常见的UI组件,它通常用于显示一些临时信息或者提供快捷操作,比如聊天气泡、提示信息等,本文将介绍如何在Android中实现一个可触摸移动的悬浮窗。
创建悬浮窗服务
我们需要创建一个继承自Service
的服务类,并在其中创建和控制悬浮窗。
1.1. 定义悬浮窗服务
import android.app.Service; import android.content.Intent; import android.graphics.PixelFormat; import android.os.IBinder; import android.view.Gravity; import android.view.LayoutInflater; import android.view.MotionEvent; import android.view.View; import android.view.WindowManager; import android.widget.TextView; public class FloatingWindowService extends Service { private WindowManager windowManager; private View floatingView; @Override public IBinder onBind(Intent intent) { return null; } @Override public void onCreate() { super.onCreate(); windowManager = (WindowManager) getSystemService(WINDOW_SERVICE); // Inflate the custom view from XML layout floatingView = LayoutInflater.from(this).inflate(R.layout.floating_window, null); // Set the layout parameters for the window WindowManager.LayoutParams params = new WindowManager.LayoutParams( WindowManager.LayoutParams.WRAP_CONTENT, WindowManager.LayoutParams.WRAP_CONTENT, WindowManager.LayoutParams.TYPE_APPLICATION_OVERLAY, WindowManager.LayoutParams.FLAG_NOT_FOCUSABLE, PixelFormat.TRANSLUCENT); params.gravity = Gravity.TOP | Gravity.LEFT; params.x = 0; params.y = 100; // Add the view to the window manager windowManager.addView(floatingView, params); // Set touch listener for the floating view floatingView.setOnTouchListener(new View.OnTouchListener() { private int initialX; private int initialY; private float initialTouchX; private float initialTouchY; @Override public boolean onTouch(View v, MotionEvent event) { switch (event.getAction()) { case MotionEvent.ACTION_DOWN: initialX = params.x; initialY = params.y; initialTouchX = event.getRawX(); initialTouchY = event.getRawY(); return true; case MotionEvent.ACTION_UP: return true; case MotionEvent.ACTION_MOVE: params.x = initialX + (int) (event.getRawX() initialTouchX); params.y = initialY + (int) (event.getRawY() initialTouchY); windowManager.updateViewLayout(floatingView, params); return true; } return false; } }); } @Override public void onDestroy() { super.onDestroy(); if (floatingView != null) windowManager.removeView(floatingView); } }
1.2. 配置权限和服务声明
在AndroidManifest.xml
文件中添加以下内容:
<uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW"/> <application> ... <service android:name=".FloatingWindowService" /> </application>
创建悬浮窗布局文件
我们需要创建一个自定义的悬浮窗布局文件,在res/layout/floating_window.xml
中定义如下内容:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/floating_layout" android:layout_width="wrap_content" android:layout_height="wrap_content" android:background="#FF0000" <!-设置背景颜色 --> android:padding="10dp" android:orientation="vertical"> <TextView android:id="@+id/text_view" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="悬浮窗" android:textColor="#FFFFFF" /> </LinearLayout>
启动悬浮窗服务
在需要启动悬浮窗的地方,例如在主Activity中,调用悬浮窗服务:
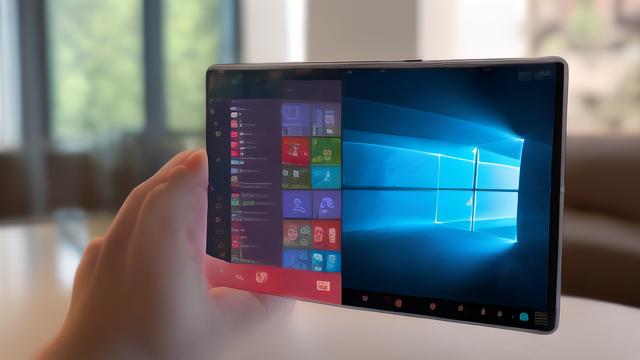
Intent serviceIntent = new Intent(this, FloatingWindowService.class); startService(serviceIntent);
通过以上步骤,我们已经实现了一个简单的可触摸移动的悬浮窗,以下是一些需要注意的事项:
1、权限问题:由于悬浮窗涉及到系统级权限,需要在应用的权限管理中手动授予SYSTEM_ALERT_WINDOW
权限,对于Android 6.0及以上版本,还需要动态申请权限。
2、性能优化:悬浮窗可能会影响用户体验,因此要谨慎使用,并尽量减少其对性能的影响。
3、兼容性:不同版本的Android系统对悬浮窗的支持可能有所不同,需要进行相应的适配和测试。
4、安全性:悬浮窗可能会被恶意利用,因此在设计时需要考虑安全性问题。
5、用户体验:悬浮窗的设计应简洁明了,避免干扰用户的正常使用。
各位小伙伴们,我刚刚为大家分享了有关“Android实现触摸移动的悬浮窗”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!