ASP(Active Server Pages)是一种服务器端脚本技术,广泛用于构建动态网页和Web应用程序,本文将探讨ASP在语音应用中的使用,特别是如何通过ASP实现语音识别和语音合成功能。
一、ASP简介
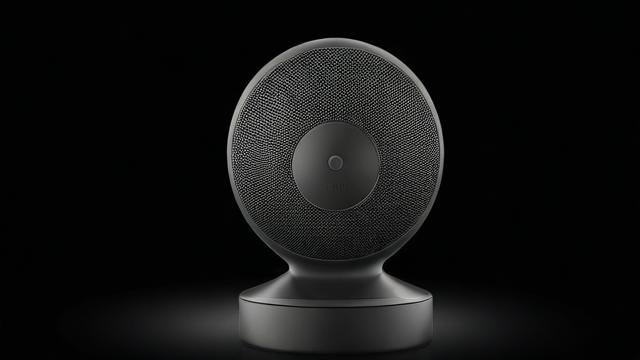
ASP是由微软开发的一种服务器端脚本语言,允许开发人员创建动态网页和Web应用程序,ASP文件通常包含HTML、CSS和JavaScript代码,以及ASP特有的脚本代码,这些代码在服务器上执行并生成客户端浏览器可以显示的内容。
二、语音识别与语音合成
语音识别(Speech Recognition)是指将人类的语音转化为计算机可以理解的文本或命令的技术,语音合成(Text-to-Speech, TTS)则是指将文本转化为人类可听懂的语音的技术,这两种技术在许多领域都有广泛的应用,如智能助手、客户服务系统和无障碍技术等。
三、ASP与语音识别
要在ASP中实现语音识别功能,通常需要借助第三方API或服务,以下是一个简单的示例,展示如何使用Google Cloud Speech-to-Text API在ASP中实现语音识别:
<%@ Language="VBScript" %> <!DOCTYPE html> <html> <head> <title>语音识别示例</title> </head> <body> <form action="recognize.asp" method="post" enctype="multipart/form-data"> <input type="file" name="audioFile" /> <input type="submit" value="识别" /> </form> </body> </html> <% Sub ProcessRequest() Dim audioFile, filePath, responseText audioFile = Request.Form("audioFile") filePath = Server.MapPath("uploads/") & "audio.wav" audioFile.SaveAs filePath ' 调用Google Cloud Speech-to-Text API responseText = CallGoogleCloudAPI(filePath) Response.Write "识别结果: " & responseText End Sub Function CallGoogleCloudAPI(filePath) Dim url, http, jsonResponse url = "https://speech.googleapis.com/v1/speech:recognize?key=YOUR_API_KEY" Set http = Server.CreateObject("MSXML2.ServerXMLHTTP.6.0") http.Open "POST", url, False http.setRequestHeader "Content-Type", "application/json" ' 构建请求体 Dim requestBody requestBody = "{" & vbCrLf & _ " ""config"": {" & vbCrLf & _ " ""encoding"": ""LINEAR16"", " & vbCrLf & _ " ""sampleRateHertz"": 16000," & vbCrLf & _ " ""languageCode"": ""en-US"" " & vbCrLf & _ " }," & vbCrLf & _ " ""audio"": {" & vbCrLf & _ " ""uri"": ""gs://YOUR_BUCKET/" & filePath & """" & vbCrLf & _ " }" & vbCrLf & _ "}" http.send requestBody jsonResponse = http.responseText CallGoogleCloudAPI = jsonResponse End Function %>
在这个示例中,用户可以通过表单上传音频文件,服务器端脚本会将文件保存到指定路径,并通过Google Cloud Speech-to-Text API进行语音识别,最后将识别结果显示在页面上。
四、ASP与语音合成
同样地,要在ASP中实现语音合成功能,也可以使用第三方API或服务,以下是一个使用Google Cloud Text-to-Speech API的示例:
<%@ Language="VBScript" %> <!DOCTYPE html> <html> <head> <title>语音合成示例</title> </head> <body> <form action="synthesize.asp" method="post"> <textarea name="text" rows="4" cols="50"></textarea><br /> <input type="submit" value="合成" /> </form> </body> </html> <% Sub ProcessRequest() Dim text, responseText text = Request.Form("text") ' 调用Google Cloud Text-to-Speech API responseText = CallGoogleCloudTTSAPI(text) Response.ContentType = "audio/mp3" Response.AddHeader "Content-Disposition", "attachment; filename=synthesized_voice.mp3" Response.BinaryWrite responseText End Sub Function CallGoogleCloudTTSAPI(text) Dim url, http, jsonResponse, audioData url = "https://texttospeech.googleapis.com/v1/text:synthesize?key=YOUR_API_KEY" Set http = Server.CreateObject("MSXML2.ServerXMLHTTP.6.0") http.Open "POST", url, False http.setRequestHeader "Content-Type", "application/json" ' 构建请求体 Dim requestBody requestBody = "{" & vbCrLf & _ " ""input"": {" & vbCrLf & _ " ""text"": "" & Server.URLEncode(text) & """", " & vbCrLf & _ " ""languageCode"": ""en-US"" " & vbCrLf & _ " }," & vbCrLf & _ " ""voice"": {" & vbCrLf & _ " ""languageCode"": ""en-US"", " & vbCrLf & _ " ""ssmlGender"": ""NEUTRAL"" " & vbCrLf & _ " }," & vbCrLf & _ " ""audioConfig"": {" & vbCrLf & _ " ""audioEncoding"": ""MP3"" " & vbCrLf & _ " }" & vbCrLf & _ "}" http.send requestBody jsonResponse = http.responseText ' 解析响应并获取音频数据 Dim jsonObj, audioContentUrl, audioDataUrl Set jsonObj = JSON.parse(jsonResponse) audioContentUrl = jsonObj("audioContent").ToString() ' 下载音频数据 Set http = Server.CreateObject("MSXML2.ServerXMLHTTP.6.0") http.Open "GET", audioContentUrl, False http.send audioData = http.responseBody CallGoogleCloudTTSAPI = audioData End Function %>
在这个示例中,用户可以输入文本,服务器端脚本会通过Google Cloud Text-to-Speech API将文本转化为语音,并将生成的音频文件作为附件返回给用户。
通过ASP结合第三方API,可以轻松实现语音识别和语音合成功能,这种方法不仅简化了开发过程,还能利用现有的成熟技术提供高质量的语音处理能力,无论是构建智能对话系统还是提升用户体验,ASP都是一个很好的选择。
FAQs

Q1: 如何在ASP中使用Google Cloud Speech-to-Text API进行语音识别?
A1: 要使用Google Cloud Speech-to-Text API进行语音识别,首先需要设置一个表单让用户上传音频文件,在服务器端脚本中,读取上传的音频文件并将其保存到服务器上的指定路径,构建请求体并调用Google Cloud Speech-to-Text API,将音频文件发送到API进行处理,解析API返回的JSON响应,提取识别出的文本并显示在页面上,具体实现可以参考上述代码示例。
Q2: 如何在ASP中使用Google Cloud Text-to-Speech API进行语音合成?
A2: 要使用Google Cloud Text-to-Speech API进行语音合成,首先需要设置一个表单让用户输入要合成的文本,在服务器端脚本中,读取用户输入的文本并构建请求体,调用Google Cloud Text-to-Speech API,将文本发送到API进行处理,API会返回一个包含音频数据的URL,再次调用该URL下载音频数据,将音频数据作为附件返回给用户,具体实现可以参考上述代码示例。
到此,以上就是小编对于“asp 语音”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。