MapReduce复合Key与复合查询
MapReduce是一种用于处理和生成大规模数据集的编程模型,它将计算任务分布到多个节点上进行处理,本文将详细介绍如何在MapReduce中使用复合Key和进行复合查询,并探讨其实现方法和应用场景。
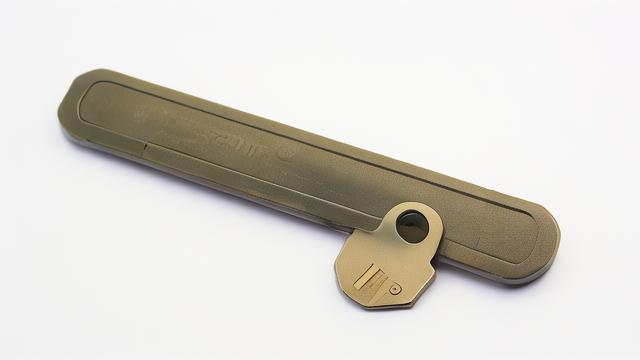
一、复合Key的定义和使用
在MapReduce中,复合Key指的是由多个字段组合而成的键,这种键可以有效地对数据进行分组和排序,从而实现更复杂的数据处理需求,复合Key通常用于需要基于多个维度进行数据分析的场景。
1. 复合Key的组成
复合Key通常由多个字段组合而成,这些字段可以是表的不同列或不同的数据属性,在一个电商网站的数据中,复合Key可以由用户ID和商品ID组成,以统计每个用户购买每种商品的次数。
2. 复合Key的实现
在MapReduce中,复合Key的实现主要通过自定义WritableComparable接口来完成,以下是一个简单的示例:
public class CompositeKey implements WritableComparable<CompositeKey> { private int userID; private int productID; // 构造函数、getter和setter略 @Override public void write(DataOutput out) throws IOException { out.writeInt(userID); out.writeInt(productID); } @Override public void readFields(DataInput in) throws IOException { userID = in.readInt(); productID = in.readInt(); } @Override public int compareTo(CompositeKey o) { int result = Integer.compare(this.userID, o.userID); if (result == 0) { result = Integer.compare(this.productID, o.productID); } return result; } @Override public int hashCode() { return Objects.hash(userID, productID); } @Override public boolean equals(Object obj) { if (this == obj) return true; if (obj == null || getClass() != obj.getClass()) return false; CompositeKey that = (CompositeKey) obj; return userID == that.userID && productID == that.productID; } @Override public String toString() { return userID + "\t" + productID; } }
二、复合查询的定义和应用
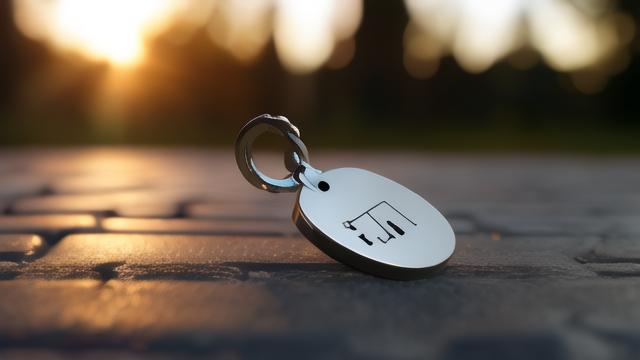
复合查询指的是在一个查询操作中涉及多个条件或多个表的连接查询,在MapReduce中,复合查询可以通过多个Mapper和Reducer的组合来实现。
1. 复合查询的类型
多条件查询:在单个表中根据多个条件进行过滤和分组,统计每个用户在不同时间段内的购买次数。
多表连接查询:在多个表之间进行连接操作,统计每个用户购买的商品的总金额。
2. 复合查询的实现
以下是一个使用MapReduce实现多表连接查询的示例:
假设有两个表:订单表(orders)和用户表(users),结构如下:
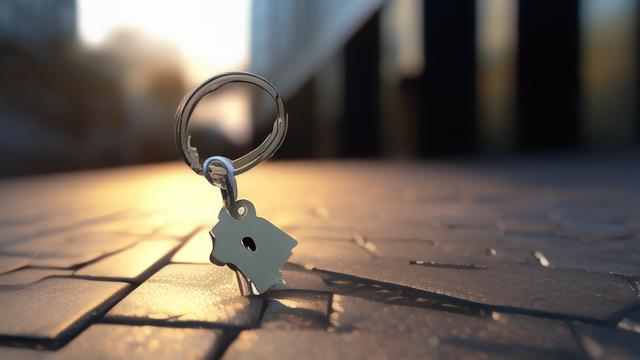
orders: order_id, user_id, product_id, amount users: user_id, name, age
目标是统计每个用户的总购买金额。
Mapper阶段:读取订单表和用户表的数据,并生成中间键值对。
public class OrderMapper extends Mapper<LongWritable, Text, CompositeKey, IntWritable> { private CompositeKey compositeKey = new CompositeKey(); private IntWritable amount = new IntWritable(0); @Override protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException { String[] fields = value.toString().split("\t"); compositeKey.setUserID(Integer.parseInt(fields[1])); compositeKey.setProductID(Integer.parseInt(fields[2])); amount.set(Integer.parseInt(fields[3])); context.write(compositeKey, amount); } }
Reducer阶段:根据用户ID进行分组,并计算每个用户的总购买金额。
public class TotalAmountReducer extends Reducer<CompositeKey, IntWritable, CompositeKey, IntWritable> { @Override protected void reduce(CompositeKey key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException { int totalAmount = 0; for (IntWritable val : values) { totalAmount += val.get(); } context.write(key, new IntWritable(totalAmount)); } }
三、复合Key和复合查询的结合使用
在实际的大数据处理场景中,复合Key和复合查询往往结合使用,以实现更复杂的数据分析需求,在一个电商平台中,统计每个用户在不同时间段内购买不同商品的总金额。
1. 复合Key的设计
可以将用户ID、时间区间和商品ID组合成一个复合Key,以便在MapReduce过程中进行分组和聚合。
2. 复合查询的实现
通过多个Mapper和Reducer的组合,实现数据的过滤、连接和聚合,具体实现可以参考上述多表连接查询的示例。
MapReduce中的复合Key和复合查询是处理大规模数据的有力工具,通过合理设计和实现复合Key,可以实现多维度的数据分组和排序;通过多个Mapper和Reducer的组合,可以实现复杂的多条件和多表连接查询,在实际应用中,复合Key和复合查询的结合使用可以满足各种复杂的数据分析需求,提高数据处理的效率和准确性。
到此,以上就是小编对于“mapreduce复合key_复合查询”的问题就介绍到这了,希望介绍的几点解答对大家有用,有任何问题和不懂的,欢迎各位朋友在评论区讨论,给我留言。