Linux 异步通知
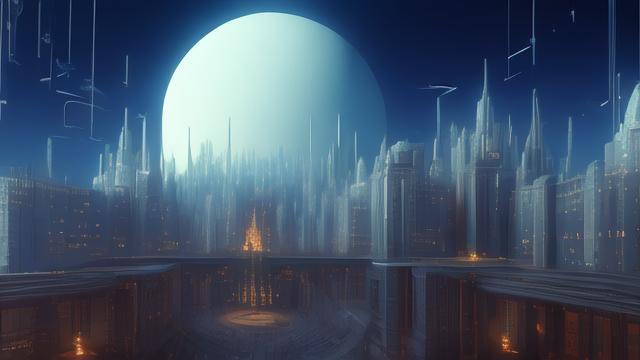
在Linux系统中,异步通知(Asynchronous Notification)是一种机制,用于在事件或条件发生时,及时地通知应用程序,这种机制可以用于多种场景,如文件系统监控、网络通信、定时任务等,本文将详细介绍Linux异步通知的概念、类型、实现方式以及常见问题解答。
1. 异步通知的概念
异步通知是指在事件发生时,系统主动向应用程序发送通知,而不是让应用程序不断轮询以检查事件是否发生,这种方式可以提高程序的效率,减少资源消耗。
2. 异步通知的类型
Linux提供了多种异步通知机制,主要包括以下几种:
1. Signals
信号是Linux中最基本的异步通知机制,信号是一种软件中断,用于通知进程发生了某种事件,常见的信号包括SIGINT(中断信号)、SIGTERM(终止信号)等。
2. inotify
inotify是一种文件系统事件监控机制,可以监控文件系统的变化,如文件的创建、删除、修改等。
3. poll和select
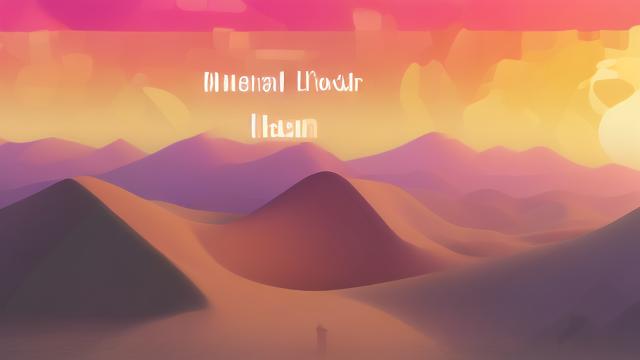
poll和select是两种用于监控文件描述符状态的系统调用,可以监控多个文件描述符,当其中某个文件描述符的状态发生变化时,返回相应的事件。
4. epoll
epoll是对poll的改进,它可以监控大量的文件描述符,并且性能更高。
2.5. timer_create和timer_settime
这两个系统调用用于创建和设置定时器,当定时器到期时,会向进程发送SIGALRM信号。
3. 异步通知的实现方式
1. 使用signal处理异步通知
#include <stdio.h> #include <stdlib.h> #include <signal.h> #include <unistd.h> void handle_signal(int sig) { printf("Received signal %d ", sig); } int main() { signal(SIGINT, handle_signal); while (1) { printf("Waiting for signal... "); sleep(1); } return 0; }
3.2. 使用inotify监控文件系统变化
#include <stdio.h> #include <stdlib.h> #include <sys/inotify.h> #include <unistd.h> int main() { int fd = inotify_init(); if (fd < 0) { perror("inotify_init"); exit(EXIT_FAILURE); } int wd = inotify_add_watch(fd, "/path/to/directory", IN_MODIFY | IN_CREATE | IN_DELETE); if (wd == -1) { perror("inotify_add_watch"); exit(EXIT_FAILURE); } while (1) { char buffer[1024]; int length = read(fd, buffer, sizeof(buffer)); if (length < 0) { perror("read"); exit(EXIT_FAILURE); } int i = 0; while (i < length) { struct inotify_event *event = (struct inotify_event *)&buffer[i]; if (event->len) { if (event->mask & IN_CREATE) { printf("File %s was created. ", event->name); } else if (event->mask & IN_DELETE) { printf("File %s was deleted. ", event->name); } else if (event->mask & IN_MODIFY) { printf("File %s was modified. ", event->name); } } i += sizeof(struct inotify_event) + event->len; } } inotify_rm_watch(fd, wd); close(fd); return 0; }
3.3. 使用epoll监控多个文件描述符
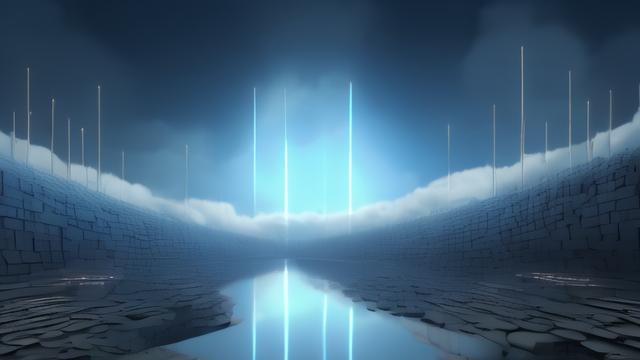
#include <stdio.h> #include <stdlib.h> #include <sys/epoll.h> #include <fcntl.h> #include <unistd.h> #include <string.h> int main() { int epoll_fd = epoll_create1(0); if (epoll_fd == -1) { perror("epoll_create1"); exit(EXIT_FAILURE); } int fd = open("/dev/input/event0", O_RDONLY); if (fd == -1) { perror("open"); exit(EXIT_FAILURE); } struct epoll_event event; event.events = EPOLLIN; event.data.fd = fd; if (epoll_ctl(epoll_fd, EPOLL_CTL_ADD, fd, &event) == -1) { perror("epoll_ctl"); exit(EXIT_FAILURE); } while (1) { struct epoll_event events[10]; int n = epoll_wait(epoll_fd, events, 10, -1); for (int i = 0; i < n; i++) { if (events[i].events & EPOLLIN) { char buffer[256]; int length = read(events[i].data.fd, buffer, sizeof(buffer)); if (length > 0) { printf("Read %d bytes from %d ", length, events[i].data.fd); } } } } close(fd); close(epoll_fd); return 0; }
4. 使用定时器处理异步通知
#include <stdio.h> #include <stdlib.h> #include <signal.h> #include <time.h> #include <unistd.h> void handle_alarm(int sig) { printf("Alarm signal received "); } int main() { signal(SIGALRM, handle_alarm); timer_t timerid; struct itimerspec its; its.it_value.tv_sec = 5; // 初始延迟5秒 its.it_value.tv_nsec = 0; its.it_interval.tv_sec = 5; // 每隔5秒触发一次 its.it_interval.tv_nsec = 0; if (timer_create(CLOCK_REALTIME, NULL, &timerid) == -1) { perror("timer_create"); exit(EXIT_FAILURE); } if (timer_settime(timerid, 0, &its, NULL) == -1) { perror("timer_settime"); exit(EXIT_FAILURE); } while (1) { pause(); // 等待信号 } return 0; }
4. 常见问题解答(FAQs)
Q1: 如何捕获并处理信号?
A1: 在Linux中,可以使用signal
函数来设置信号处理函数,要捕获并处理SIGINT信号,可以使用以下代码:
#include <stdio.h> #include <stdlib.h> #include <signal.h> #include <unistd.h> void handle_signal(int sig) { printf("Received signal %d ", sig); } int main() { signal(SIGINT, handle_signal); while (1) { printf("Waiting for signal... "); sleep(1); } return 0; }
在这个例子中,我们使用signal
函数将SIGINT信号的处理函数设置为handle_signal
,当接收到SIGINT信号时,handle_signal
函数将被调用。
Q2: 如何使用inotify监控文件系统变化?
A2: 使用inotify监控文件系统变化需要以下几个步骤:
1、初始化inotify实例。
2、添加需要监控的文件或目录。
3、循环读取事件并处理。
4、清理资源。
下面是一个示例代码:
#include <stdio.h> #include <stdlib.h> #include <sys/inotify.h> #include <unistd.h> int main() { int fd = inotify_init(); if (fd < 0) { perror("inotify_init"); exit(EXIT_FAILURE); } int wd = inotify_add_watch(fd, "/path/to/directory", IN_MODIFY | IN_CREATE | IN_DELETE); if (wd == -1) { perror("inotify_add_watch"); exit(EXIT_FAILURE); } while (1) { char buffer[1024]; int length = read(fd, buffer, sizeof(buffer)); if (length < 0) { perror("read"); exit(EXIT_FAILURE); } int i = 0; while (i < length) { struct inotify_event *event = (struct inotify_event *)&buffer[i]; if (event->len) { if (event->mask & IN_CREATE) { printf("File %s was created. ", event->name); } else if (event->mask & IN_DELETE) { printf("File %s was deleted. ", event->name); } else if (event->mask & IN_MODIFY) { printf("File %s was modified. ", event->name); } } i += sizeof(struct inotify_event) + event->len; } } inotify_rm_watch(fd, wd); close(fd); return 0; }
小伙伴们,上文介绍了“linux 异步通知”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。