c
命令通常指的是 cc
或 gcc
,用于编译 C 语言程序。Linux C 路径操作详解
目录结构与文件路径
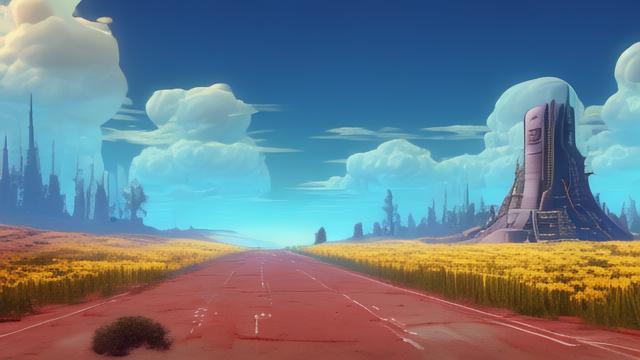
Linux中的文件系统采用层次化的树状目录结构,每个目录都可以包含文件或其他子目录,了解并掌握Linux文件路径的相关知识对于编写程序和操作系统都非常重要。
绝对路径与相对路径
绝对路径:从文件系统的根目录开始,完整地指定一个文件或目录的路径。/home/user/file.txt
,这种路径表示方法是唯一的,无论当前工作目录是什么。
相对路径:相对于当前工作目录的路径。Documents/file.txt
,这种路径表示方法依赖于当前的工作目录。
获取文件名和路径
在C语言中,可以使用标准库函数来获取文件名和路径。
提取文件名
头文件:<libgen.h>

char *basename(char *path);
该函数返回指向最后一个斜杠( 提取路径 头文件: 该函数返回指向最后一个斜杠之前的字符部分,即路径,注意,这个函数会修改传入的 示例代码 输出结果: 可以看到, 在Linux系统中,有多种方式可以获取当前工作目录。 头文件: 该函数获取当前工作目录,并将结果存储在 头文件: 该函数读取符号链接的目标路径,结合 Q1:如何在C语言中获取文件的绝对路径? A1:在C语言中,可以使用 Q2:如何更改C语言程序的头文件搜索路径? A2:可以通过设置环境变量 或者在编译时使用 各位小伙伴们,我刚刚为大家分享了有关“c 路径 linux”的知识,希望对你们有所帮助。如果您还有其他相关问题需要解决,欢迎随时提出哦!/
)之后的字符部分,即文件名,注意,这个函数不会修改传入的path
<libgen.h>
char *dirname(char *path);
path
内容,如果不想改变原path
,需要重新申请一个缓冲区。
#include <stdio.h>
#include <libgen.h>
#include <string.h>
#include <stdlib.h>
static char s_filepath[] = "/mnt/usr/file.txt";
int main(int argc, char **argv) {
char *path;
printf("FILE_PATH FILE : %s
", basename(s_filepath));
printf("1 -s_filepath = %s
", s_filepath);
path = strdup(s_filepath);
printf("1 -path = %s
", path);
printf("FILE_PATH PATH : %s
", dirname(path));
printf("2 -path = %s
", path);
free(path);
return 0;
}
FILE_PATH FILE : file.txt
1 -s_filepath = /mnt/usr/file.txt
1 -path = /mnt/usr/file.txt
FILE_PATH PATH : /mnt/usr
2 -path = /mnt/us
dirname
函数修改了传入的path
获取当前工作目录
getcwd
函数<unistd.h>
char *getcwd(char *buf, size_t size);
buf
中,需要确保buf
的大小足够大以容纳路径。readlink
函数<unistd.h>
ssize_t readlink(const char *path, char *buf, size_t bufsiz);
/proc/self/exe
可以获取目标程序所在目录。
#include <limits.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <errno.h>
void pr_currendir() {
char buf[PATHNAME_MAX];
// 使用getcwd获取启动目录
if (NULL == getcwd(buf, sizeof(buf))) {
fprintf(stderr, "getcwd error: %s
", strerror(errno));
exit(1);
}
printf("current work path: %s
", buf);
char *s;
// 使用get_current_dir_name获取启动目录
if ((s = get_current_dir_name()) == NULL) {
fprintf(stderr, "getcwd error: %s
", strerror(errno));
exit(1);
}
printf("current dir name: %s
", s);
if (s != NULL) {
free(s); // 别忘了free get_current_dir_name malloc的缓冲区
}
}
常见问题解答(FAQs)
realpath
函数将相对路径转换为绝对路径,头文件为<limits.h>
和<stdlib.h>
,示例如下:
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
int main() {
char abs_path[PATH_MAX];
char *relative_path = "Documents/file.txt";
if (realpath(relative_path, abs_path) != NULL) {
printf("Absolute path: %s
", abs_path);
} else {
perror("Error getting absolute path");
}
return 0;
}
C_INCLUDE_PATH
来更改C语言程序的头文件搜索路径,可以在shell中使用以下命令:
export C_INCLUDE_PATH=/new/include/path:$C_INCLUDE_PATH
-I
选项指定头文件路径:
gcc -I/new/include/path your_program.c -o your_program