Negate: Understanding and Applying the Logical Operation
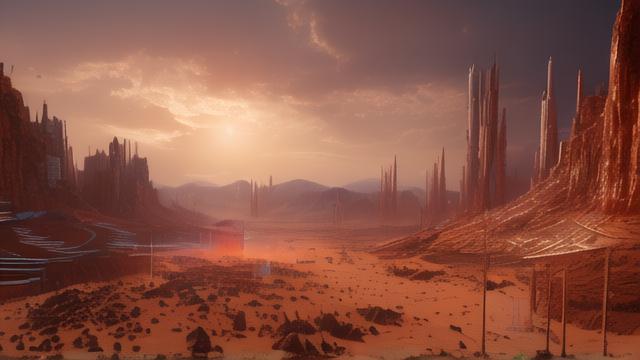
What is Negate?
Negate, in the context of logic and computing, refers to the operation that inverts a boolean value. In other words, it changestrue
tofalse
andfalse
totrue
. This operation is fundamental in digital electronics, computer science, and mathematics. The negation operation is typically represented by the symbol¬
(the tilde or "not" symbol) or sometimes by an exclamation mark!
in programming languages.
Basic Concepts of Negate
Boolean Algebra
In Boolean algebra, negate is a unary operation that affects a single operand. The basic rules are as follows:
Negation of true (¬1
):¬1 = 0
Negation of false (¬0
):¬0 = 1
These can be summarized in a truth table:
Operand | Negate (¬) |
0 | 1 |
1 | 0 |
Digital Electronics
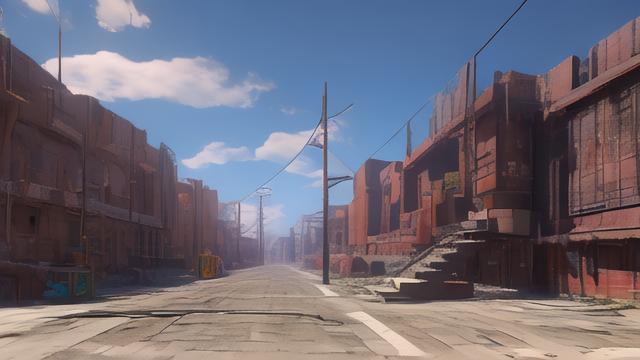
In digital electronics, negation is implemented using logic gates. The NOT gate (often symbolized as a triangle or an inverted triangle) performs the negation operation. For instance:
If the input to a NOT gate is high (1), the output will be low (0).
If the input to a NOT gate is low (0), the output will be high (1).
Programming Languages
In many programming languages, the negation operator is used to invert boolean expressions. Here are some examples:
C/C++:!true
evaluates tofalse
, and!false
evaluates totrue
.
Python:not True
evaluates toFalse
, andnot False
evaluates toTrue
.
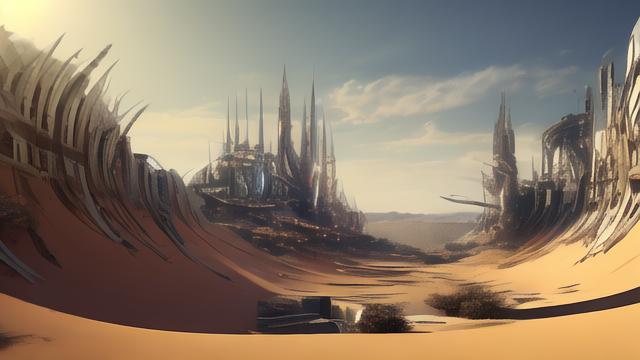
JavaScript:!true
evaluates tofalse
, and!false
evaluates totrue
.
Applications of Negate
Control Flow in Programming
The negation operator is frequently used in control flow statements such asif
,while
, andfor
loops. For example:
is_raining = False if not is_raining: print("Let's go for a walk.") else: print("Stay indoors.")
In this case, sinceis_raining
isFalse
,not is_raining
isTrue
, so the message "Let's go for a walk." is printed.
Logic Gates in Digital Circuits
Negation is essential in constructing complex digital circuits. By combining NOT gates with AND, OR, and other logic gates, engineers can create circuits that perform various logical operations and computations.
Error Handling
In software development, negation can play a crucial role in error handling. For instance, checking if a file did not open successfully:
try: file = open('example.txt') except FileNotFoundError: print("File not found.")
Here, the absence of an exception indicates success, while the presence of an exception indicates failure.
Advanced Topics in Negate
De Morgan's Theorems
De Morgan's Theorems provide a way to negate compound logical expressions. They state:
¬(A ∧ B) = (¬A) ∨ (¬B)
¬(A ∨ B) = (¬A) ∧ (¬B)
These theorems allow you to push the negation inside the parentheses and change the operators accordingly.
Double Negation
Double negation (!!A
or!!!A
) results in the original value. This concept is useful in simplifying expressions and understanding logical equivalences.
double_negation = not not True # This evaluates to True triple_negation = not not not True # This also evaluates to True
Negation in Fuzzy Logic
In fuzzy logic, negation can take on a range of values between 0 and 1 rather than being strictly binary. This allows for more nuanced decision-making in systems that require degrees of truth.
Practical Examples in Code
Here are practical examples demonstrating the use of negation in different programming scenarios:
Example 1: Simple Conditional Check
is_logged_in = False if not is_logged_in: print("Please log in.")
Output:Please log in.
Example 2: Loop until a Condition is Met
max_attempts = 3 attempts = 0 while not attempts >= max_attempts: print("Trying again...") attempts += 1
Output: The loop will print "Trying again..." three times before exiting.
Example 3: Error Handling with Negation
file_path = 'non_existent_file.txt' try: with open(file_path) as file: content = file.read() except FileNotFoundError: print("File not found.")
Output:File not found.
because the file does not exist.
FAQs
Q1: What is the difference between logical negation and bitwise negation?
A1: Logical negation inverts the boolean value (i.e., changestrue
tofalse
and vice versa), whereas bitwise negation inverts each bit of the operand. For example:
Logical negation oftrue
results infalse
.
Bitwise negation of the binary number0001
results in1110
(assuming 4-bit representation).
Q2: Can I use negation in SQL queries?
A2: Yes, negation can be used in SQL queries, typically within theWHERE
clause to filter out records that do not meet certain conditions. For example:
SELECT * FROM users WHERE NOT active;
This query selects all users who are not active.
小伙伴们,上文介绍了“negate”的内容,你了解清楚吗?希望对你有所帮助,任何问题可以给我留言,让我们下期再见吧。